Routing
Building a route
To build a route on the map, you need to create two objects: a TrafficRouter object to find an optimal route and a RouteMapObjectSource data source to display the route on the map.
To find a route between two points, call the findRoute() method, specifying the coordinates of the start points and the end points as RouteSearchPoint objects. You can additionally specify route parameters (RouteSearchOptions) and a list of intermediate points of the route (a list of RouteSearchPoint objects).
import 'package:dgis_mobile_sdk_full/dgis.dart' as sdk;
final routeSearchOptions = sdk.RouteSearchOptions.car(sdk.CarRouteSearchOptions());
final startPoint = sdk.RouteSearchPoint(coordinates: GeoPoint(latitude: 55.759909, longitude: 37.618806));
final finishPoint = sdk.RouteSearchPoint(coordinates: GeoPoint(latitude: 55.752425, longitude: 37.613983));
final trafficRouter = sdk.TrafficRouter(sdkContext);
final routesFuture = trafficRouter.findRoute(startPoint, finishPoint, routeSearchOptions);
The call returns a deferred result with a list of TrafficRoute objects. To display the found route on the map, you need to use these objects to create RouteMapObject objects and add them to a RouteMapObjectSource data source.
import 'package:dgis_mobile_sdk_full/dgis.dart' as sdk;
// Create a data source
final sdk.RouteMapObjectSource routeMapObjectSource = sdk.RouteMapObjectSource(sdkContext, RouteVisualizationType.NORMAL)
map.addSource(routeMapObjectSource)
// Find a route
final trafficRouter = sdk.TrafficRouter(sdkContext);
final List<sdk.TrafficRoute> routes = await trafficRouter.findRoute(startSearchPoint, finishSearchPoint).value;
// After receiving the route, add it to the map
int routeIdx = 0;
bool isActive = true;
routes.forEach((sdk.TrafficRoute route) {
routeMapObjectSource.addObject(sdk.RouteMapObject(route, isActive, routeIdx));
routeIdx += 1;
isActive = false;
})
Instead of using TrafficRouter and RouteMapObjectSource objects and manually processing a list of TrafficRoute objects, you can use RouteEditor and RouteEditorSource objects. In that case, you can simply pass the coordinates of the route as a RouteEditorRouteParams object to the setRouteParams() method. The route displays automatically.
final routeEditor = sdk.RouteEditor(sdkContext);
final routeEditorSource = sdk.RouteEditorSource(sdkContext, routeEditor);
map.addSource(routeEditorSource);
routeEditor.setRouteParams(
sdk.RouteEditorRouteParams(
startPoint: startPoint,
finishPoint: finishPoint,
routeSearchOptions: routeSearchOptions,
),
);
Getting route points
To build a route, you need to get GeoPoint points, which are used to create a RouteSearchPoint object. One of the ways to do this is described below.
Getting coordinates of an object at the tap spot
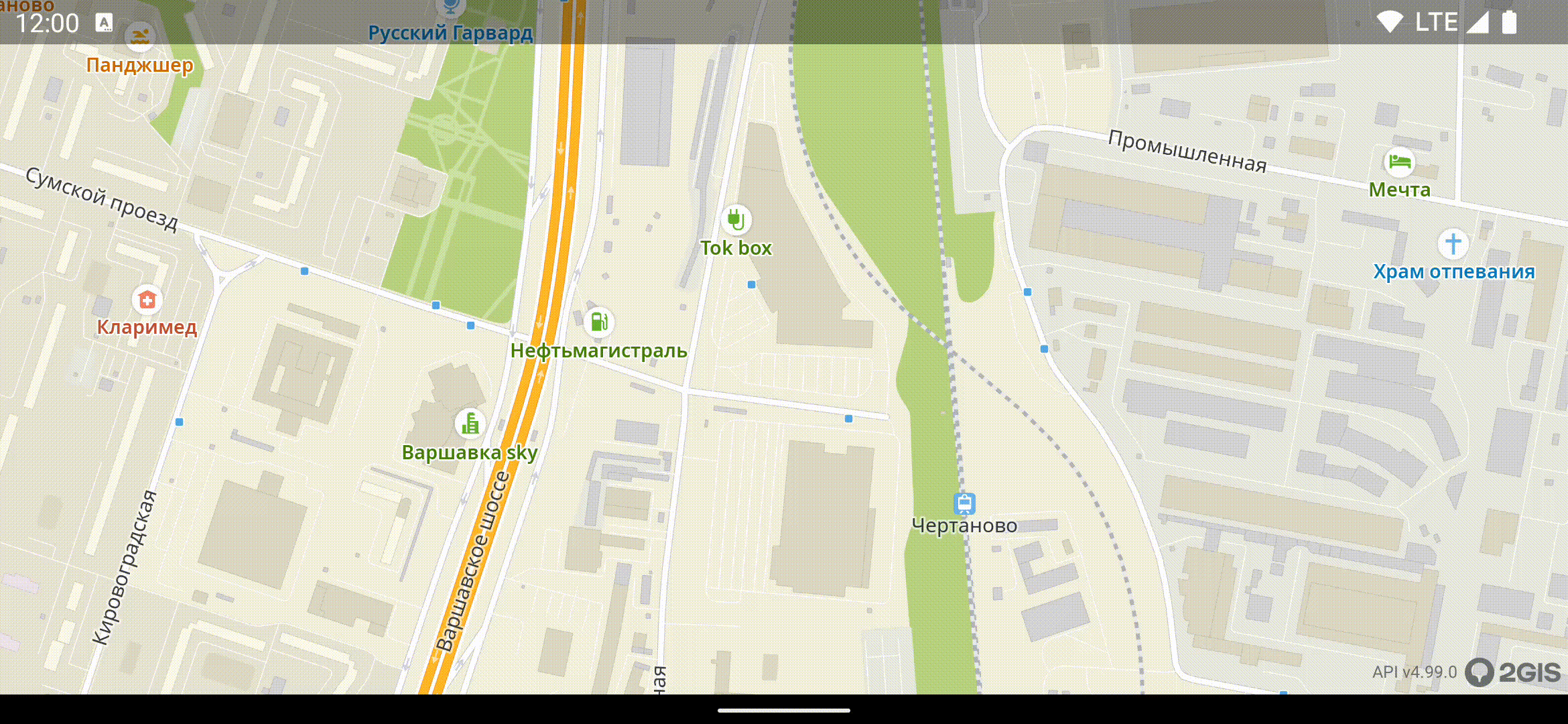
You can get the coordinates of the object (e.g., building, road) at the place where the user taps on the map. Use the getRenderedObjects() method to get a DgisMapObject object with an id
field. This identifier is a stable numeric identifier of the object directory that can help you perform a search query and get information about the object, including the geographical coordinates of its center.
import 'package:dgis_mobile_sdk_map/dgis.dart' as sdk;
map.getRenderedObjects(sdk.ScreenPoint()).then((List<sdk.RenderedObjectInfo> info) {
// Get the closest object to the tap spot inside the specified radius
final sdk.RenderedObject obj = info.first.item;
// In this example, we want to search for information about the selected object in the directory.
// To do this, make sure that the type of this object can be found
if (obj.source is sdk.DgisSource && obj.item is sdk.DgisMapObject) {
final source = obj.source as sdk.DgisSource;
// Search
final foundObject =
await sdk.SearchManager.createOnlineManager(sdkContext)
.searchByDirectoryObjectId((obj.item as sdk.DgisMapObject).id)
.value;
final sdk.GeoPoint? geoPoint = foundObject.markerPosition?.point;
}
});
Important
Coordinates of a center of a large object (for example, long and complicated ring roads) can be located far away from the camera viewport as the received object does not contain information on the camera location or the map tap spot. In such cases, use other methods to get coordinates.
Passing intermediate points
For a route with intermediate points, a user is expected to visit each point in the defined order. If an intermediate point is missed, a route may be rebuilt:
- If the GPS data shows that the user is moving not in the direction of the expected intermediate point, the route is rebuilt relatively to the current user location so that they visit the missed point. When the visit of the intermediate point is detected, the route continues.
- If the moment of visiting the intermediate point is not detected due to a weak GPS signal but later the user location is detected on the route or next to it towards the next point, the previous intermediate point is considered visited and the route continues.