Globe
You can configure the look of the map so that it turns into the globe when you zoom out far enough. If the globe mode is enabled, it works on the 2-8 zoom levels. You can configure the globe mode depending on the graphics preset and then enable it using API.
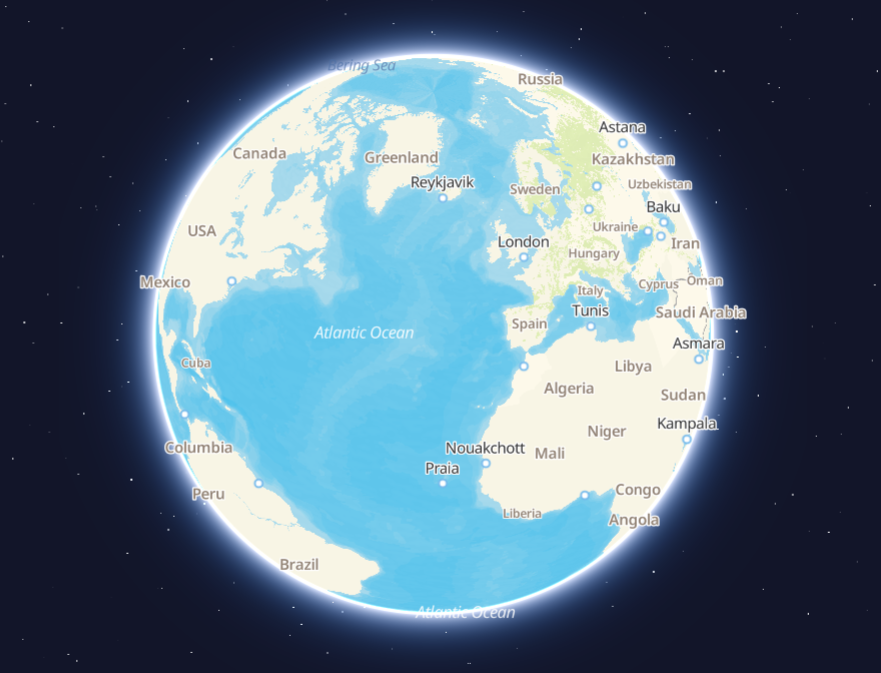
Configuring graphics presets
If you use multiple map graphics presets, it is recommended to enable the globe only in the immersive
mode, because the globe rendering requires significant system resources.
-
Open the Style editor.
-
Open the required style.
-
Configure the globe mode for the graphics preset:
-
In the Other tab, select Graphics presets:
-
Use the Globe toggle in the required presets.
-
-
Check the look of the globe in the updated graphic presets:
- Go to the Settings section.
- Enable the Globe option.
- Select the required preset from the Graphics presets drop-down list.
- Zoom out far enough to see the globe.
Enabling the globe
By default, globe mode is disabled, and you will see a flat map when you zoom out. To enable it, use the globeEnabled
global style variable during map initialization:
const map = new mapgl.Map('container', {
center,
zoom: 3,
key: 'Your API access key',
styleState: { globeEnabled: true },
});
To toggle the globe mode dynamically, use the map.patchStyleState()
method, for example:
function toggleGlobe() {
let { globeEnabled } = map.getStyleState();
map.patchStyleState({ globeEnabled: !globeEnabled });
}
Example of the map with the globe mode and a function to toggle it dynamically:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>2GIS Map API - Globe</title>
<meta name="description" content="Simple Globe for MapGL JS API" />
<style>
html,
body,
#container {
margin: 0;
width: 100%;
height: 100%;
overflow: hidden;
}
#controls {
position: absolute;
top: 10px;
left: 10px;
z-index: 2;
}
</style>
</head>
<body>
<div id="controls">
<button onclick="toggleGlobe()">Toggle Globe</button>
</div>
<div id="container"></div>
<script src="https://mapgl.2gis.com/api/js/v1"></script>
<script>
const center = [0, 25.23584];
const map = new mapgl.Map('container', {
center,
zoom: 3,
key: 'Your API access key',
// Globe is enabled via `globeEnabled` style variable
styleState: { globeEnabled: true }
});
// You can switch globe mode dynamically via `map.patchStyleState(...)` method.
function toggleGlobe () {
let { globeEnabled } = map.getStyleState();
map.patchStyleState({ globeEnabled: !globeEnabled });
}
map.setCenter([center[0] + 179, center[1]], { duration: 120000 });
</script>
</body>
</html>